Advanced PHP String Functions
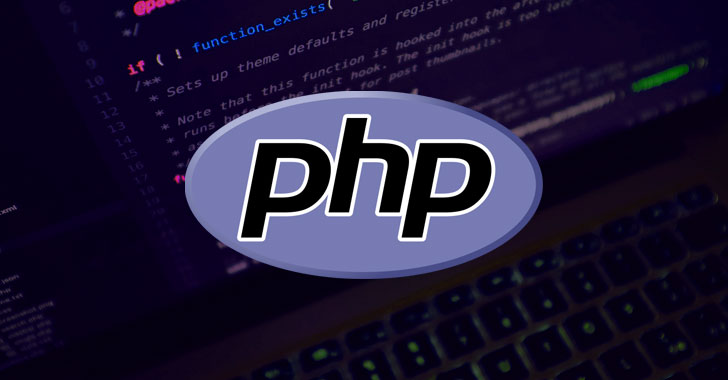
Introduction
PHP provides a wide range of string functions that help manipulate and analyze text data. This article will cover advanced string functions that are useful for various text processing tasks, complete with examples and explanations to illustrate their use.
1. str_replace() Function
Description: The str_replace() function replaces all occurrences of the search string with the replacement string.
Example:
$text = "Hello World";
$newText = str_replace("World", "PHP", $text);
echo $newText; // Output: Hello PHP
2. str_ireplace() Function
Description: The str_ireplace() function is similar to str_replace() but is case-insensitive.
Example:
$text = "Hello World";
$newText = str_ireplace("world", "PHP", $text);
echo $newText; // Output: Hello PHP
3. strpos() Function
Description: The strpos() function finds the position of the first occurrence of a substring in a string.
Example:
$text = "Hello World";
$position = strpos($text, "World");
echo $position; // Output: 6
4. strrpos() Function
Description: The strrpos() function finds the position of the last occurrence of a substring in a string.
Example:
$text = "Hello World World";
$position = strrpos($text, "World");
echo $position; // Output: 12
5. substr() Function
Description: The substr() function returns a part of a string.
Example:
$text = "Hello World";
$part = substr($text, 6, 5);
echo $part; // Output: World
6. strtoupper() Function
Description: The strtoupper() function converts all characters in a string to uppercase.
Example:
$text = "Hello World";
$upperText = strtoupper($text);
echo $upperText; // Output: HELLO WORLD
7. strtolower() Function
Description: The strtolower() function converts all characters in a string to lowercase.
Example:
$text = "Hello World";
$lowerText = strtolower($text);
echo $lowerText; // Output: hello world
8. ucwords() Function
Description: The ucwords() function capitalizes the first letter of each word in a string.
Example:
$text = "hello world";
$capitalized = ucwords($text);
echo $capitalized; // Output: Hello World
9. ucfirst() Function
Description: The ucfirst() function capitalizes the first letter of a string.
Example:
$text = "hello world";
$capitalized = ucfirst($text);
echo $capitalized; // Output: Hello world
10. lcfirst() Function
Description: The lcfirst() function makes the first character of a string lowercase.
Example:
$text = "Hello World";
$lowercaseFirst = lcfirst($text);
echo $lowercaseFirst; // Output: hello World
11. str_repeat() Function
Description: The str_repeat() function repeats a string a specified number of times.
Example:
$text = "Hello ";
$repeated = str_repeat($text, 3);
echo $repeated; // Output: Hello Hello Hello
12. str_split() Function
Description: The str_split() function splits a string into an array.
Example:
$text = "Hello";
$array = str_split($text);
print_r($array); // Output: ["H", "e", "l", "l", "o"]
13. str_shuffle() Function
Description: The str_shuffle() function randomly shuffles all characters in a string.
Example:
$text = "Hello";
$shuffled = str_shuffle($text);
echo $shuffled; // Output: Randomly shuffled "Hello"
14. trim() Function
Description: The trim() function removes whitespace or other predefined characters from both sides of a string.
Example:
$text = " Hello World ";
$trimmed = trim($text);
echo $trimmed; // Output: Hello World
15. ltrim() Function
Description: The ltrim() function removes whitespace or other predefined characters from the left side of a string.
Example:
$text = " Hello World";
$leftTrimmed = ltrim($text);
echo $leftTrimmed; // Output: Hello World
16. rtrim() Function
Description: The rtrim() function removes whitespace or other predefined characters from the right side of a string.
Example:
$text = "Hello World ";
$rightTrimmed = rtrim($text);
echo $rightTrimmed; // Output: Hello World
17. str_word_count() Function
Description: The str_word_count() function counts the number of words in a string.
Example:
$text = "Hello World";
$wordCount = str_word_count($text);
echo $wordCount; // Output: 2
18. strrev() Function
Description: The strrev() function reverses a string.
Example:
$text = "Hello";
$reversed = strrev($text);
echo $reversed; // Output: olleH
19. strcmp() Function
Description: The strcmp() function compares two strings (case-sensitive).
Example:
$text1 = "Hello";
$text2 = "hello";
$result = strcmp($text1, $text2);
echo $result; // Output: -1 (because 'H' < 'h')
20. strcasecmp() Function
Description: The strcasecmp() function compares two strings (case-insensitive).
Example:
$text1 = "Hello";
$text2 = "hello";
$result = strcasecmp($text1, $text2);
echo $result; // Output: 0 (because 'Hello' and 'hello' are considered equal)
21. substr_count() Function
Description: The substr_count() function counts the number of times a substring occurs in a string.
Example:
$text = "Hello Hello Hello";
$count = substr_count($text, "Hello");
echo $count; // Output: 3
22. strstr() Function
Description: The strstr() function finds the first occurrence of a string inside another string and returns the rest of the string.
Example:
$text = "Hello World";
$found = strstr($text, "World");
echo $found; // Output: World
23. stristr() Function
Description: The stristr() function finds the first occurrence of a case-insensitive string inside another string and returns the rest of the string.
Example:
$text = "Hello World";
$found = stristr($text, "world");
echo $found; // Output: World
24. strtok() Function
Description: The strtok() function splits a string into tokens.
Example:
$text = "Hello World PHP";
$token = strtok($text, " ");
while ($token !== false) {
echo $token . "\n";
$token = strtok(" ");
}
// Output: Hello \n World \n PHP
25. addslashes() Function
Description: The addslashes() function adds backslashes before certain characters to escape them.
Example:
$text = "Hello 'World'";
$escaped = addslashes($text);
echo $escaped; // Output: Hello \'World\'
26. stripslashes() Function
Description: The stripslashes() function removes backslashes added by addslashes().
Example:
$text = "Hello \'World\'";
$unescaped = stripslashes($text);
echo $unescaped; // Output: Hello 'World'
27. htmlspecialchars() Function
Description: The htmlspecialchars() function converts special characters to HTML entities.
Example:
$text = "Hello ";
$html = htmlspecialchars($text);
echo $html; // Output: Hello <World>
28. html_entity_decode() Function
Description: The html_entity_decode() function converts HTML entities back to their corresponding characters.
Example:
$text = "Hello <World>";
$decoded = html_entity_decode($text);
echo $decoded; // Output: Hello
29. nl2br() Function
Description: The nl2br() function inserts HTML line breaks before all newlines in a string.
Example:
$text = "Hello\nWorld";
$html = nl2br($text);
echo $html; // Output: Hello
World
30. wordwrap() Function
Description: The wordwrap() function wraps a string to a given number of characters.
Example:
$text = "Hello World, this is a long string.";
$wrapped = wordwrap($text, 10);
echo $wrapped;
// Output: Hello World,
// this is a
// long string.
31. str_pad() Function
Description: The str_pad() function pads a string to a certain length with another string.
Example:
$text = "Hello";
$padded = str_pad($text, 10, "-");
echo $padded; // Output: Hello-----
32. strtr() Function
Description: The strtr() function translates specific characters in a string.
Example:
$text = "Hello World";
$trans = strtr($text, "HW", "hw");
echo $trans; // Output: hello world
33. setlocale() Function
Description: The setlocale() function sets locale information for the program.
Example:
setlocale(LC_ALL, 'fr_FR');
echo strftime("%A"); // Output: the current day in French
34. localeconv() Function
Description: The localeconv() function returns locale information for numeric formatting.
Example:
$locale = localeconv();
print_r($locale);
// Output: array with locale-specific information
35. strtr() Function (Translation Table)
Description: The strtr() function can also use a translation table to translate multiple characters.
Example:
$text = "Hello World";
$trans = strtr($text, array("H" => "h", "W" => "w"));
echo $trans; // Output: hello world
36. strtok() Function (Tokenize with Multiple Delimiters)
Description: The strtok() function can tokenize a string using multiple delimiters.
Example:
$text = "Hello, World; PHP";
$token = strtok($text, ", ;");
while ($token !== false) {
echo $token . "\n";
$token = strtok(", ;");
}
// Output: Hello \n World \n PHP
37. implode() Function
Description: The implode() function joins array elements with a string.
Example:
$array = array("Hello", "World", "PHP");
$text = implode(" ", $array);
echo $text; // Output: Hello World PHP
38. explode() Function
Description: The explode() function splits a string into an array by a delimiter.
Example:
$text = "Hello World PHP";
$array = explode(" ", $text);
print_r($array); // Output: ["Hello", "World", "PHP"]
39. str_contains() Function
Description: The str_contains() function checks if a string contains a given substring (available in PHP 8.0+).
Example:
$text = "Hello World";
$contains = str_contains($text, "World");
echo $contains ? "Yes" : "No"; // Output: Yes
40. str_starts_with() Function
Description: The str_starts_with() function checks if a string starts with a given substring (available in PHP 8.0+).
Example:
$text = "Hello World";
$startsWith = str_starts_with($text, "Hello");
echo $startsWith ? "Yes" : "No"; // Output: Yes
41. str_ends_with() Function
Description: The str_ends_with() function checks if a string ends with a given substring (available in PHP 8.0+).
Example:
$text = "Hello World";
$endsWith = str_ends_with($text, "World");
echo $endsWith ? "Yes" : "No"; // Output: Yes
42. str_split() Function (With Length)
Description: The str_split() function can also split a string into chunks of a specified length.
Example:
$text = "HelloWorld";
$chunks = str_split($text, 5);
print_r($chunks); // Output: ["Hello", "World"]
43. mb_strtoupper() Function
Description: The mb_strtoupper() function converts a string to uppercase while considering multibyte characters.
Example:
$text = "こんにちは";
$upperText = mb_strtoupper($text);
echo $upperText; // Output: こんにちは (Japanese characters are not affected)
44. mb_strtolower() Function
Description: The mb_strtolower() function converts a string to lowercase while considering multibyte characters.
Example:
$text = "こんにちは";
$lowerText = mb_strtolower($text);
echo $lowerText; // Output: こんにちは (Japanese characters are not affected)
45. mb_substr() Function
Description: The mb_substr() function returns part of a string, while handling multibyte character encodings.
Example:
$text = "こんにちは世界";
$part = mb_substr($text, 0, 5);
echo $part; // Output: こんにちは
46. mb_strlen() Function
Description: The mb_strlen() function gets the length of a string, while handling multibyte character encodings.
Example:
$text = "こんにちは世界";
$length = mb_strlen($text);
echo $length; // Output: 7 (number of characters in Japanese)
47. preg_match() Function
Description: The preg_match() function performs a regular expression match.
Example:
$text = "Hello 123";
if (preg_match("/\d+/", $text)) {
echo "Match found!";
} else {
echo "No match.";
}
// Output: Match found!
48. preg_replace() Function
Description: The preg_replace() function performs a regular expression search and replace.
Example:
$text = "Hello 123";
$newText = preg_replace("/\d+/", "456", $text);
echo $newText; // Output: Hello 456
49. preg_split() Function
Description: The preg_split() function splits a string by a regular expression.
Example:
$text = "Hello, World; PHP";
$array = preg_split("/[,;] /", $text);
print_r($array); // Output: ["Hello", "World", "PHP"]
50. preg_match_all() Function
Description: The preg_match_all() function performs a global regular expression match.
Example:
$text = "Hello 123 World 456";
preg_match_all("/\d+/", $text, $matches);
print_r($matches[0]); // Output: ["123", "456"]