Comprehensive Guide to PHP Array Functions
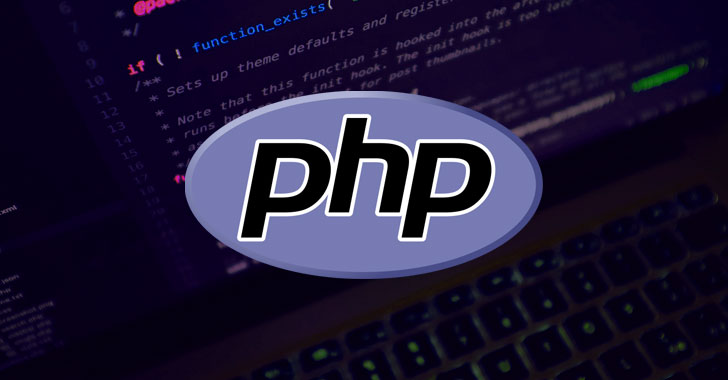
Introduction
PHP provides a rich set of functions to manipulate arrays, which are one of the most commonly used data structures in programming. This article covers 50 essential PHP array functions with practical examples, from basic to advanced levels, to help you efficiently manage and process data in your applications.
1. array() Function
Description: The array() function is used to create an array in PHP.
Example:
$fruits = array("Apple", "Banana", "Orange");
print_r($fruits);
2. count() Function
Description: The count() function returns the number of elements in an array.
Example:
$fruits = array("Apple", "Banana", "Orange");
echo count($fruits); // Output: 3
3. array_push() Function
Description: The array_push() function adds one or more elements to the end of an array.
Example:
$fruits = array("Apple", "Banana");
array_push($fruits, "Orange", "Grape");
print_r($fruits); // Output: ["Apple", "Banana", "Orange", "Grape"]
4. array_pop() Function
Description: The array_pop() function removes the last element from an array.
Example:
$fruits = array("Apple", "Banana", "Orange");
$last = array_pop($fruits);
echo $last; // Output: Orange
print_r($fruits); // Output: ["Apple", "Banana"]
5. array_shift() Function
Description: The array_shift() function removes the first element from an array.
Example:
$fruits = array("Apple", "Banana", "Orange");
$first = array_shift($fruits);
echo $first; // Output: Apple
print_r($fruits); // Output: ["Banana", "Orange"]
6. array_unshift() Function
Description: The array_unshift() function adds one or more elements to the beginning of an array.
Example:
$fruits = array("Banana", "Orange");
array_unshift($fruits, "Apple", "Grape");
print_r($fruits); // Output: ["Apple", "Grape", "Banana", "Orange"]
7. array_slice() Function
Description: The array_slice() function extracts a portion of an array.
Example:
$fruits = array("Apple", "Banana", "Orange", "Grape");
$sliced = array_slice($fruits, 1, 2);
print_r($sliced); // Output: ["Banana", "Orange"]
8. array_splice() Function
Description: The array_splice() function removes a portion of an array and optionally replaces it with other elements.
Example:
$fruits = array("Apple", "Banana", "Orange", "Grape");
array_splice($fruits, 1, 2, array("Kiwi", "Mango"));
print_r($fruits); // Output: ["Apple", "Kiwi", "Mango", "Grape"]
9. array_keys() Function
Description: The array_keys() function returns all the keys of an array.
Example:
$fruits = array("a" => "Apple", "b" => "Banana", "c" => "Orange");
$keys = array_keys($fruits);
print_r($keys); // Output: ["a", "b", "c"]
10. array_values() Function
Description: The array_values() function returns all the values of an array.
Example:
$fruits = array("a" => "Apple", "b" => "Banana", "c" => "Orange");
$values = array_values($fruits);
print_r($values); // Output: ["Apple", "Banana", "Orange"]
11. array_merge() Function
Description: The array_merge() function merges one or more arrays.
Example:
$array1 = array("Apple", "Banana");
$array2 = array("Orange", "Grape");
$merged = array_merge($array1, $array2);
print_r($merged); // Output: ["Apple", "Banana", "Orange", "Grape"]
12. array_diff() Function
Description: The array_diff() function compares array values and returns the differences.
Example:
$array1 = array("Apple", "Banana", "Orange");
$array2 = array("Banana", "Grape");
$diff = array_diff($array1, $array2);
print_r($diff); // Output: ["Apple", "Orange"]
13. array_intersect() Function
Description: The array_intersect() function compares array values and returns the matches.
Example:
$array1 = array("Apple", "Banana", "Orange");
$array2 = array("Banana", "Grape", "Orange");
$intersect = array_intersect($array1, $array2);
print_r($intersect); // Output: ["Banana", "Orange"]
14. array_flip() Function
Description: The array_flip() function exchanges all keys with their associated values in an array.
Example:
$array = array("a" => "Apple", "b" => "Banana");
$flipped = array_flip($array);
print_r($flipped); // Output: ["Apple" => "a", "Banana" => "b"]
15. array_unique() Function
Description: The array_unique() function removes duplicate values from an array.
Example:
$array = array("Apple", "Banana", "Apple", "Orange");
$unique = array_unique($array);
print_r($unique); // Output: ["Apple", "Banana", "Orange"]
16. array_sort() Function
Description: The array_sort() function sorts an array in ascending order.
Example:
$array = array("Banana", "Apple", "Orange");
sort($array);
print_r($array); // Output: ["Apple", "Banana", "Orange"]
17. rsort() Function
Description: The rsort() function sorts an array in descending order.
Example:
$array = array("Banana", "Apple", "Orange");
rsort($array);
print_r($array); // Output: ["Orange", "Banana", "Apple"]
18. asort() Function
Description: The asort() function sorts an array and maintains index association.
Example:
$array = array("a" => "Banana", "b" => "Apple", "c" => "Orange");
asort($array);
print_r($array); // Output: ["b" => "Apple", "a" => "Banana", "c" => "Orange"]
19. ksort() Function
Description: The ksort() function sorts an array by key in ascending order.
Example:
$array = array("b" => "Banana", "a" => "Apple", "c" => "Orange");
ksort($array);
print_r($array); // Output: ["a" => "Apple", "b" => "Banana", "c" => "Orange"]
20. krsort() Function
Description: The krsort() function sorts an array by key in descending order.
Example:
$array = array("b" => "Banana", "a" => "Apple", "c" => "Orange");
krsort($array);
print_r($array); // Output: ["c" => "Orange", "b" => "Banana", "a" => "Apple"]
21. array_reverse() Function
Description: The array_reverse() function returns an array with elements in reverse order.
Example:
$array = array("Apple", "Banana", "Orange");
$reversed = array_reverse($array);
print_r($reversed); // Output: ["Orange", "Banana", "Apple"]
22. array_search() Function
Description: The array_search() function searches for a value in an array and returns the corresponding key.
Example:
$array = array("a" => "Apple", "b" => "Banana", "c" => "Orange");
$key = array_search("Banana", $array);
echo $key; // Output: b
23. in_array() Function
Description: The in_array() function checks if a value exists in an array.
Example:
$array = array("Apple", "Banana", "Orange");
$exists = in_array("Banana", $array);
echo $exists ? 'true' : 'false'; // Output: true
24. array_merge_recursive() Function
Description: The array_merge_recursive() function merges arrays recursively.
Example:
$array1 = array("a" => array("Apple"), "b" => array("Banana"));
$array2 = array("a" => array("Orange"), "c" => array("Grape"));
$merged = array_merge_recursive($array1, $array2);
print_r($merged); // Output: ["a" => ["Apple", "Orange"], "b" => ["Banana"], "c" => ["Grape"]]
25. array_combine() Function
Description: The array_combine() function creates an array by using one array for keys and another for values.
Example:
$keys = array("a", "b", "c");
$values = array("Apple", "Banana", "Orange");
$combined = array_combine($keys, $values);
print_r($combined); // Output: ["a" => "Apple", "b" => "Banana", "c" => "Orange"]
26. array_fill() Function
Description: The array_fill() function fills an array with a specified value.
Example:
$array = array_fill(0, 3, "Apple");
print_r($array); // Output: ["Apple", "Apple", "Apple"]
27. array_fill_keys() Function
Description: The array_fill_keys() function fills an array with a specified value using the keys from another array.
Example:
$keys = array("a", "b", "c");
$array = array_fill_keys($keys, "Apple");
print_r($array); // Output: ["a" => "Apple", "b" => "Apple", "c" => "Apple"]
28. array_map() Function
Description: The array_map() function applies a callback function to the elements of the given arrays.
Example:
$numbers = array(1, 2, 3, 4);
$squared = array_map(function($num) {
return $num * $num;
}, $numbers);
print_r($squared); // Output: [1, 4, 9, 16]
29. array_filter() Function
Description: The array_filter() function filters elements of an array using a callback function.
Example:
$numbers = array(1, 2, 3, 4, 5);
$evenNumbers = array_filter($numbers, function($num) {
return $num % 2 == 0;
});
print_r($evenNumbers); // Output: [2, 4]
30. array_reduce() Function
Description: The array_reduce() function iteratively reduces the array to a single value using a callback function.
Example:
$numbers = array(1, 2, 3, 4);
$sum = array_reduce($numbers, function($carry, $item) {
return $carry + $item;
}, 0);
echo $sum; // Output: 10
31. array_walk() Function
Description: The array_walk() function applies a user-defined function to each element of an array.
Example:
$fruits = array("apple", "banana", "orange");
array_walk($fruits, function(&$value, $key) {
$value = ucfirst($value);
});
print_r($fruits); // Output: ["Apple", "Banana", "Orange"]
32. array_key_exists() Function
Description: The array_key_exists() function checks if the specified key exists in an array.
Example:
$array = array("a" => "Apple", "b" => "Banana");
$exists = array_key_exists("b", $array);
echo $exists ? 'true' : 'false'; // Output: true
33. array_column() Function
Description: The array_column() function returns the values from a single column in the input array.
Example:
$array = array(
array("id" => 1, "name" => "John"),
array("id" => 2, "name" => "Jane"),
array("id" => 3, "name" => "Doe")
);
$names = array_column($array, "name");
print_r($names); // Output: ["John", "Jane", "Doe"]
34. array_combine() Function
Description: The array_combine() function creates an array by using one array for keys and another for values.
Example:
$keys = array("a", "b", "c");
$values = array("Apple", "Banana", "Orange");
$combined = array_combine($keys, $values);
print_r($combined); // Output: ["a" => "Apple", "b" => "Banana", "c" => "Orange"]
35. array_multisort() Function
Description: The array_multisort() function sorts multiple arrays or a multi-dimensional array.
Example:
$array1 = array("Apple", "Banana", "Orange");
$array2 = array(3, 1, 2);
array_multisort($array2, SORT_ASC, $array1);
print_r($array1); // Output: ["Banana", "Orange", "Apple"]
print_r($array2); // Output: [1, 2, 3]
36. array_sum() Function
Description: The array_sum() function returns the sum of all the values in an array.
Example:
$numbers = array(1, 2, 3, 4);
$sum = array_sum($numbers);
echo $sum; // Output: 10
37. array_product() Function
Description: The array_product() function returns the product of all the values in an array.
Example:
$numbers = array(1, 2, 3, 4);
$product = array_product($numbers);
echo $product; // Output: 24
38. array_rand() Function
Description: The array_rand() function returns one or more random keys from an array.
Example:
$array = array("Apple", "Banana", "Orange");
$key = array_rand($array);
echo $array[$key]; // Output: Random fruit
39. array_chunk() Function
Description: The array_chunk() function splits an array into chunks.
Example:
$array = array("Apple", "Banana", "Orange", "Grape");
$chunks = array_chunk($array, 2);
print_r($chunks); // Output: [["Apple", "Banana"], ["Orange", "Grape"]]
40. array_keys() Function
Description: The array_keys() function returns all the keys of an array.
Example:
$array = array("a" => "Apple", "b" => "Banana", "c" => "Orange");
$keys = array_keys($array);
print_r($keys); // Output: ["a", "b", "c"]
41. array_values() Function
Description: The array_values() function returns all the values of an array.
Example:
$array = array("a" => "Apple", "b" => "Banana", "c" => "Orange");
$values = array_values($array);
print_r($values); // Output: ["Apple", "Banana", "Orange"]
42. array_change_key_case() Function
Description: The array_change_key_case() function changes the case of all keys in an array.
Example:
$array = array("A" => "Apple", "B" => "Banana");
$lowercase = array_change_key_case($array, CASE_LOWER);
print_r($lowercase); // Output: ["a" => "Apple", "b" => "Banana"]
43. array_intersect_key() Function
Description: The array_intersect_key() function returns an array containing all the entries of the first array which have matching keys with all the other arrays.
Example:
$array1 = array("a" => "Apple", "b" => "Banana", "c" => "Orange");
$array2 = array("a" => "Avocado", "b" => "Blueberry");
$intersect = array_intersect_key($array1, $array2);
print_r($intersect); // Output: ["a" => "Apple", "b" => "Banana"]
44. array_diff_assoc() Function
Description: The array_diff_assoc() function compares arrays with additional checks for key association.
Example:
$array1 = array("a" => "Apple", "b" => "Banana");
$array2 = array("a" => "Apple", "b" => "Blueberry");
$diff = array_diff_assoc($array1, $array2);
print_r($diff); // Output: ["b" => "Banana"]
45. array_intersect_assoc() Function
Description: The array_intersect_assoc() function returns the array of values that are present in all input arrays with additional checks for key association.
Example:
$array1 = array("a" => "Apple", "b" => "Banana");
$array2 = array("a" => "Apple", "b" => "Blueberry");
$intersect = array_intersect_assoc($array1, $array2);
print_r($intersect); // Output: ["a" => "Apple"]