Top 15 PHP Interview Questions and Answers
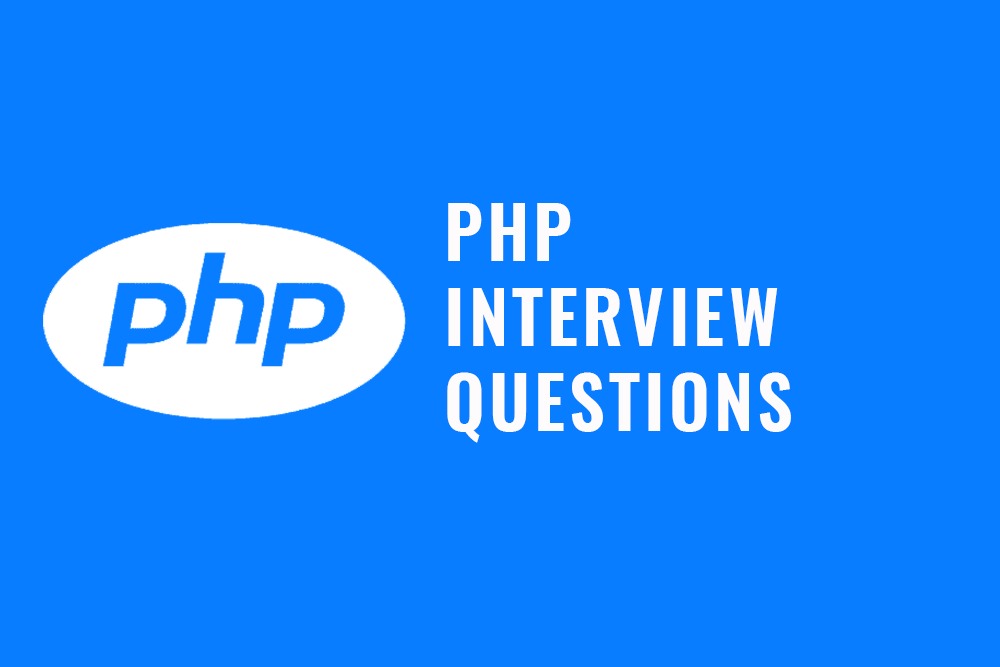
Introduction
Preparing for a PHP interview? Here are the top 15 questions you might encounter, along with detailed answers.
1. What is PHP?
PHP stands for "Hypertext Preprocessor". It is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML.
2. What are the common uses of PHP?
PHP is commonly used for:
- Server-side scripting
- Command line scripting
- Writing desktop applications
3. What is a PHP variable and how do you declare it?
A PHP variable is used to store data such as strings, numbers, arrays, and objects. You declare a variable in PHP using the $ symbol, followed by the variable name. For example:
$variable_name = "value";
4. Explain the difference between `echo` and `print` in PHP.
`echo` and `print` are both used to output data to the screen. The main differences are:
- `echo` can output multiple strings, while `print` can only output one string.
- `echo` is marginally faster than `print`.
- `print` returns a value (1), so it can be used in expressions.
5. How do you create a PHP function?
You can create a PHP function using the `function` keyword, followed by the function name, parentheses, and a code block. For example:
function myFunction() {
// code to be executed
}
6. What is an array in PHP, and how do you create one?
An array in PHP is a data structure that stores multiple values in a single variable. You can create an array using the `array()` function or the shorthand `[]` syntax. For example:
$array = array("value1", "value2", "value3");
$shorthandArray = ["value1", "value2", "value3"];
7. Explain the difference between indexed arrays and associative arrays in PHP.
Indexed arrays use numeric indices to access their values, while associative arrays use named keys. For example:
// Indexed array
$indexedArray = array("value1", "value2");
// Associative array
$associativeArray = array("key1" => "value1", "key2" => "value2");
8. How do you handle errors in PHP?
PHP provides several functions for error handling, such as `error_reporting()`, `set_error_handler()`, and `trigger_error()`. You can use try-catch blocks to handle exceptions. For example:
try {
// Code that may throw an exception
} catch (Exception $e) {
echo "Caught exception: ", $e->getMessage();
}
9. What is the difference between GET and POST methods in PHP?
`GET` method sends data via URL parameters and is limited in length, suitable for retrieving data. `POST` method sends data via HTTP headers, has no size limitations, and is suitable for sending sensitive information.
10. How do you connect to a MySQL database in PHP?
You can connect to a MySQL database using the `mysqli` or `PDO` extensions. For example, using `mysqli`:
$conn = new mysqli("servername", "username", "password", "dbname");
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
11. What are prepared statements in PHP and why are they used?
Prepared statements are used to execute the same SQL statements repeatedly with high efficiency. They help prevent SQL injection attacks. Using `PDO`:
$stmt = $conn->prepare("INSERT INTO users (name, email) VALUES (?, ?)");
$stmt->bind_param("ss", $name, $email);
$stmt->execute();
12. What is the purpose of the `header()` function in PHP?
The `header()` function sends a raw HTTP header to the client. It is used for redirection, content type specification, and controlling cache behavior. For example:
header("Location: http://www.example.com");
13. How do you manage sessions in PHP?
Sessions in PHP are managed using `session_start()`, `$_SESSION` superglobal, and `session_destroy()`. For example:
session_start();
$_SESSION["username"] = "admin";
session_destroy();
14. What is the difference between `include` and `require` in PHP?
`include` and `require` both include and evaluate a specified file, but `require` causes a fatal error if the file cannot be included, while `include` only emits a warning.
15. What is Composer in PHP?
Composer is a dependency management tool in PHP, used for managing libraries and packages required by your project. It allows you to declare the libraries your project depends on and installs them for you.